π§° Web3 [Serie Part 2/10] - Connect Web3 to Ganache
![π§° Web3 [Serie Part 2/10] - Connect Web3 to Ganache](/content/images/size/w960/2021/09/web3js-tutorial-part0-2.png)
At the end of this chapter, we will be able to connect our code to our local blockchain (Ganache), and retrieve account balance in Ether.
Introduction
In this part, we are going to configure Web3 to enable communication with Ganache, or any other nodes.
To allow interactions, Web3 is requiring a 'provider'.
What is a Web3 provider?
A provider is a data structure providing a bridge with the publicly reachable Ethereum nodes. In other words, a provider is how Web3 talks to the blockchain (more info here).
The provider actually sends the call in JSON-RPC to the Ethereum nodes. Thanks to this design, the users keep flexibility, and is able to decide what wallet he is going to use.
Usually, the Ethereum wallet exposes the provider object as a global object in Javascript. In any case, our dApp will test whether the provider is public, and if it is, we will create a Web3 instance to connect to the blockchain using the wallet provider.
Let's dive in the code
In our main directory, where we installed our dependencies, we create a file 'index.js'.
touch index.js
In this file, let's write the following code. Comments are following.
const Web3 = require('web3');
const web3 = new Web3('http://127.0.0.1:7545');
- We import the library in our code. It's important to write Web3 with an Uppercase to differentiate the Object, from the instance we create after.
- Finally, we instantiate Web3 using the RPC Service url provided by Ganache http://127.0.0.1:7545.
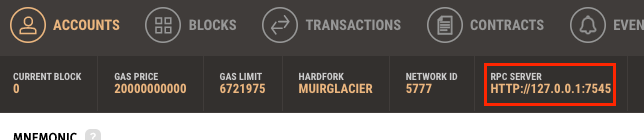
We are now connected to our local Blockchain.
Smoke Test
Now we are connected, let's test our code. We will try to retrieve the Ether Balance from 1st account generated by Ganache.
Here is the code. Comments are following.
const Web3 = require('web3');
const web3 = new Web3('http://127.0.0.1:7545');
const main = async() => {
const address = '0x8ba7dDC383130c3D5c3afC76AD3f3343571246E8';
const balance = await web3.eth.getBalance(address);
console.log(balance);
}
main();
- We define a async function called main.
- Then, we copy the first account address provided by Ganache.
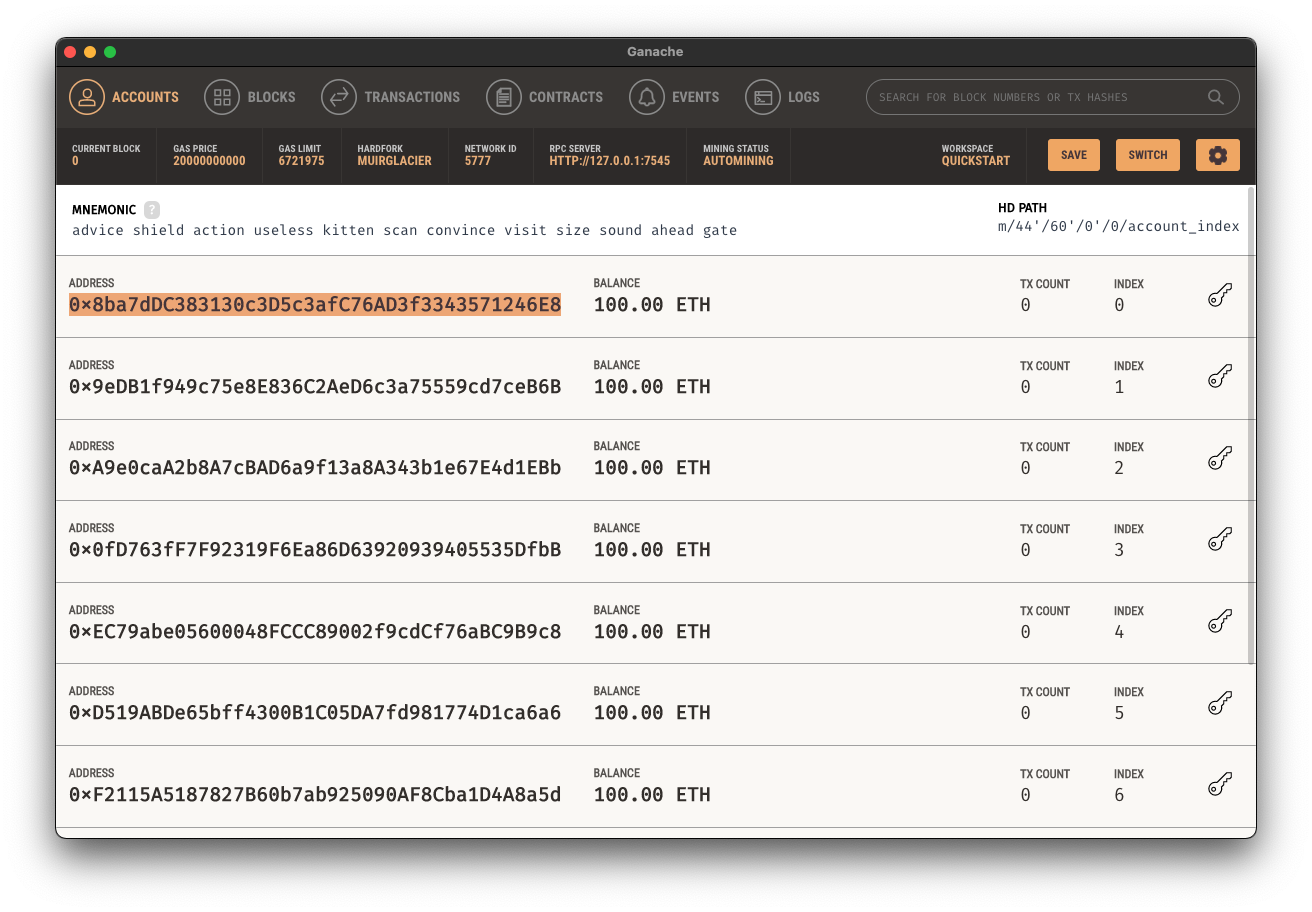
- Finally, we use the web3.eth.getBalance(address [, defaultBlock] [, callback]) method allowing us to retrieve the balance for specific account.
After launching the code, we will see the following result in the Terminal
100000000000000000000
Why donβt we see 100 ETH?
By default, the getBalance function returns the value in Wei, which corresponds to 10^-18 ETH.
We can use the 'utils' toolbox from web3 to convert this Wei amount into Eth.
const Web3 = require('web3');
const web3 = new Web3('http://127.0.0.1:7545');
const main = async() => {
const address = '0x8ba7dDC383130c3D5c3afC76AD3f3343571246E8';
const balanceWei = await web3.eth.getBalance(address);
const balanceEth = await web3.utils.fromWei(balanceWei);
console.log(balanceEth);
}
main();
After running that script, we will see 100 ETH as a result.
100
π π₯³ π―ββοΈ π Congratulations! We successfully setup our provider, and communicate with Ganache !
Let's move on to the next part.